For Dashboard Designers Parameters
Friday, March 15, 2019 9:54 PMParameters
Different Functions have different behaviours when they are executed, which can be further customized using parameters. For example, a Neo4jQuery has a cypher
parameter that tells the Function the cypher query it needs to execute. Besides predefined parameters, custom parameters can also be defined. The Function itself will not behave differently based on these custom parameters, but they can be passed on to the next Function.
Setting parameters
Parameters can be set in a few different ways:
- by a Function node property
- by a Trigger relation property
- by user input
Parameter by Function node property
The most basic method to set a Function parameter is by setting it as a node property on the Function's node, with the parameter name as the key and the desired parameter value as property value. Each time the Function is executed, it will use the provided value for its parameter.
With the plain notation, the parameter value cannot be changed unless the Function node property is changed. However, it is possible to allow parameter overriding, e.g. by an incoming Trigger or user prompt, by prefixing the node property with a $
or #
parameter prefix. The default value will stay the same, but if another value is provided, it will be overwritten.
In the example below, a very simple Neo4jQuery is presented with two parameters set as node properties:
-
name
: a fixed parameter value that cannot be overridden -
$cypher
: a cypher statement that can be overridden by incoming Triggers or user prompts
Read on to find out more about setting parameters by Triggers or user prompts.
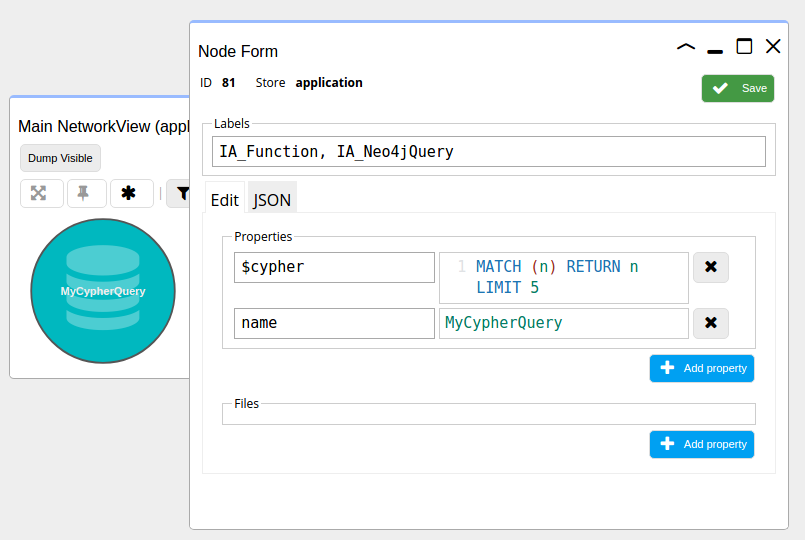
Parameter by a Trigger relation property
If a Function parameter is set with a parameter prefix ($
or #
), it can be overridden by an incoming Trigger to that Function. Similar to the Function node property, the Trigger relation property can specify the desired value for the parameter. The Trigger relation property, however, must have a $
or #
parameter prefix. Otherwise the property is considered a Trigger matching property. Through parameters, Triggers can pass data from one Function to the next.
Parameter by a user prompt
Parameters can also be provided by user input, through user prompts. In order to prompt a user for the value of a parameter before the Function is executed, a few meta parameters can be set to the parameter, indicated by a colon (:
), e.g. $cypher:prompt : "Please enter a cypher query"
. These prompt meta parameters must have a parameter prefix.
The options are:
Key | Description | Possible values |
---|---|---|
:prompt |
Text to display to ask the user for input. | Any string |
:promptDefault |
Default value to show in the input field. | Number or string |
:default |
Default value to use if no value is provided. | Any value |
:index |
Index to sort the input fields by (low to high) | Number or string |
Overriding order
With multiple ways to set and override a parameter value, the order of evaluation is important to determine the final value of the parameter. The 'weakest' method sets the value first, the 'strongest' has the last override. The order of evaluation is therefore (from weak to strong):
- Function node-defined property with parameter prefix
- Function node-defined user prompt
- Trigger relation-defined property
- Trigger relation-defined user prompt
- Function node-defined property without parameter prefix
Parameter prefix
Besides a symbol that allows parameter overrides, the parameter prefix specifies if the parameter value should be forced into single element form ($
) or list form (#
). If the value to a #
parameter is a single element, it will be converted into a list of one item. Similarly, if the value to a $
parameter is a list, only the first element will be used as the parameter value.
$_path
parameter
A special parameter that every Function has, is the $_path
parameter. In contrast to other parameters, the value of this parameter is passed automatically from Function to Function, without having to explicitly specify this. This can be used, for instance, to execute Functions based on the path through which the Function was reached. In the following example, Function A has parameters $_path.origin
and $otherParameter
set to some value. Whereas the $otherParameter
value is lost beyond Function B, the $_path.origin
value is still available at Function D.
The origin
name in this example is arbitrary, and the $_path
can contain any number of sub-properties that will be passed on from Function to Function.
Parameter evaluation
By default, parameter values are used by the receiving Functions exactly how they are. It is also possible to use
dynamic values, by entering a small code snippet that is evaluated using a basic Javascript interpreter. For example,
the value "1+1" can be evaluated as 2 in the receiving Function. The Javascript interpreter has 3 different levels of
evaluation: none
, path
and full
. This level can be set per parameter, as a meta property of the parameter.
Example:
"$number1": "1+1",
"$number2": "2+2",
"$number1:evaluate": "full"
The :evaluate
meta property on $number1
sets the evaluation level to interpret the value as a calculation.
The resulting value for $number1
will be 2
, but $number2
(without the :evaluate
meta property) will still be "2+2"
.
Parameter templating
All parameters, by default, support templating. This means that placeholders such as {{myParameter}}
will be filled in with the value of the parameter called myParameter
. For example, a Function with the following parameters:
{
"$firstName": "John",
"$lastName": "Doe",
"$fullName": "{{firstName}} {{lastName}}"
}
would fill in the values from firstName
and lastName
into fullName
, to form John Doe
.
To disable templating, set the :templating
meta property to none
:
{
"$fullName": "{{firstName}} {{lastName}}",
"$fullName:templating": "none"
}
With :templating
set to none
, fullName
would resolve to exactly {{firstName}} {{lastName}}
; the placeholders will not be replaced.
Supported statements
The 3 evaluation levels (none
, path
, full
) support an increasing number of statements. The full
level supports
most, the path
(default) level a subset of that, and none
always takes the raw value. Below are the supported statement types.
Type | Minimum level | Example | Description |
---|---|---|---|
Literal | path |
123 |
Interpreted as the number 123 |
Basic functions | path |
map({a:1, b:2}) |
Only map and flatten from Lodash. Value is the result of the function. |
MemberExpression | path |
(%).data.name |
(%) refers to a Trigger event as an object. With the dots, properties of the object can be fetched. |
Array mapping | path |
(%).nodes[#].id |
[#] fetches the next property (.id in this case) from each object in the array ((%).nodes ). |
Lodash functions | full |
max(1,2) |
Value is the result of the function. Lodash documentation can be found here. Omit the _. from the function call. |
Operators | full |
+ , - , && , || , ! |
Javascript operators. |
Array | full |
[1,2,3] |
Create an array. |
Object | full |
{name: 'John', age: 37} |
Create an object. |
Conditional | full |
(%).age > 21 ? 1 : 2 |
If the age property of the trigger event is larger than 21, this will evaluate to 1, otherwise 2. |